- Published on
Automated Testing Strategies Boosting Software Quality with Efficiency
- Authors
- Name
- Adil ABBADI
Introduction
Automated testing has become the backbone of modern software development, allowing teams to deliver reliable software at speed. Leveraging automation not only reduces manual testing efforts but ensures consistency and accuracy across every deployment. In this article, we’ll explore key automated testing strategies, highlight practical examples, and offer guidance on successfully implementing automated tests in your workflow.
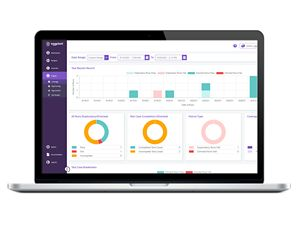
- The Testing Pyramid: Structuring Your Tests
- Automating Tests in CI/CD Pipelines
- Best Practices for Automated Testing Strategies
- Conclusion
- Ready to Automate Your Testing Pipeline?
The Testing Pyramid: Structuring Your Tests
A robust automated testing strategy often starts with the Testing Pyramid, which helps teams balance speed, coverage, and reliability by prioritizing different types of tests.
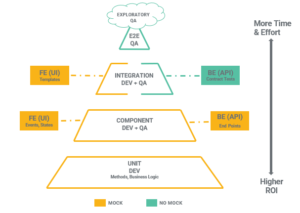
Unit Testing
Unit tests validate individual components or functions in isolation. These are fast, straightforward, and form the pyramid’s foundation.
// JavaScript (using Jest)
function add(a, b) {
return a + b;
}
test('adds 2 + 2 to equal 4', () => {
expect(add(2, 2)).toBe(4);
});
Integration Testing
Integration tests ensure multiple components work together correctly, catching issues that unit tests may miss.
// Node.js/Express integration test (using Supertest)
const request = require('supertest');
const app = require('./app');
test('POST /login authenticates user', async () => {
const response = await request(app)
.post('/login')
.send({ username: 'user', password: 'secret' });
expect(response.statusCode).toBe(200);
});
End-to-End (E2E) Testing
E2E tests simulate real user scenarios and validate the entire system’s workflow.
// Cypress E2E test
describe('Login Flow', () => {
it('successfully logs in', () => {
cy.visit('/login');
cy.get('input[name=username]').type('user');
cy.get('input[name=password]').type('password');
cy.get('button[type=submit]').click();
cy.url().should('include', '/dashboard');
});
});
Automating Tests in CI/CD Pipelines
Integrating automated tests into CI/CD pipelines ensures every code change is validated early and often, preventing buggy releases.
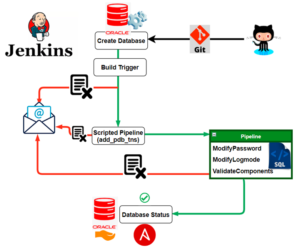
Setting Up Test Automation in CI
Most modern CI tools (like GitHub Actions, GitLab CI, Jenkins) allow you to configure automated test steps:
# .github/workflows/test.yml (GitHub Actions)
name: Test Suite
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Install dependencies
run: npm ci
- name: Run unit tests
run: npm test
Parallelizing and Optimizing Tests
Large test suites can become bottlenecks; parallelizing tests and running them in containers scale execution efficiently.
# Example: Jest parallel execution
jest --maxWorkers=4
Best Practices for Automated Testing Strategies
Developing a maintainable and scalable automated test strategy requires careful planning and discipline.
Test Maintainability
- Use descriptive test names and clear assertions.
- Refactor test code to remove duplication.
- Use setup/teardown hooks to avoid flaky tests.
Code Coverage and Quality
- Measure test coverage, but don’t equate high coverage with quality.
- Focus on critical paths in your application.
// Run Jest tests with code coverage report
npm test -- --coverage
Continuous Feedback and Reporting
- Integrate test reports and notifications with your CI tool.
- Fail the build on critical test failures and alert the team promptly.
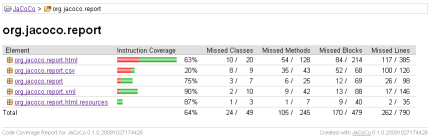
Conclusion
Automated testing is an essential ingredient for high-quality, resilient software. Structuring your strategy using the Testing Pyramid, integrating tests into CI/CD, and following best practices amplifies your team’s productivity and confidence in every release.
Ready to Automate Your Testing Pipeline?
Start small, automate early, and iterate on your test strategy. With careful design and the right tools, automated testing can be your strongest ally on the path to software excellence!