- Published on
Building High-Performance APIs with Python FastAPI A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
APIs power modern web and mobile applications, and Python’s FastAPI framework is revolutionizing how developers build them. Known for its blazing speed, ease-of-use, and robust type system, FastAPI is rapidly becoming the go-to choice for both startups and large enterprises. In this comprehensive guide, we’ll dive into FastAPI development—from getting started to implementing asynchronous endpoints—unlocking best practices for building performant, scalable APIs.
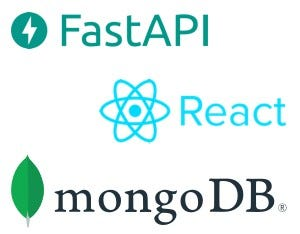
- Setting Up FastAPI for a New Project
- Creating Endpoints and Handling Requests
- Embracing Asynchronous Programming
- Essential Tips and Best Practices
- Conclusion
- Ready to Build Your Next API?
Setting Up FastAPI for a New Project
Starting with FastAPI is refreshingly simple. Before diving into code, you’ll need Python 3.7+ and pip installed on your system.
# Install FastAPI and an ASGI server (Uvicorn recommended)
pip install fastapi uvicorn
Next, create your project directory and your first FastAPI application:
# filename: main.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def root():
return {"message": "Welcome to FastAPI!"}
To run your app, execute:
uvicorn main:app --reload
Visit http://localhost:8000
in your browser to see your running API. FastAPI also auto-generates interactive docs at /docs
and /redoc
.
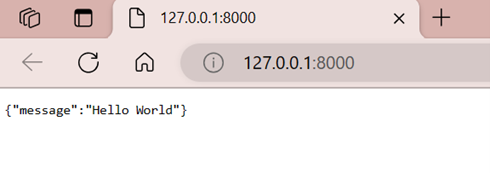
Creating Endpoints and Handling Requests
One of FastAPI’s core strengths is its intuitive endpoint creation, leveraging Python’s type hints for data validation and documentation.
Here’s how to create endpoints for retrieving, adding, and deleting items in a mock to-do list:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import List
app = FastAPI()
class Todo(BaseModel):
id: int
task: str
completed: bool = False
todos: List[Todo] = []
@app.post("/todos/", response_model=Todo)
async def create_todo(todo: Todo):
todos.append(todo)
return todo
@app.get("/todos/", response_model=List[Todo])
async def get_todos():
return todos
@app.delete("/todos/{todo_id}", response_model=dict)
async def delete_todo(todo_id: int):
for i, todo in enumerate(todos):
if todo.id == todo_id:
todos.pop(i)
return {"result": "Todo deleted"}
raise HTTPException(status_code=404, detail="Todo not found")
- Automatic Docs: Visit
/docs
for Swagger UI (interactive API docs) and/redoc
for ReDoc-style docs, all auto-generated from your code and type hints. - Validation & Serialization: Pydantic models ensure incoming data is validated and serialized properly before processing.
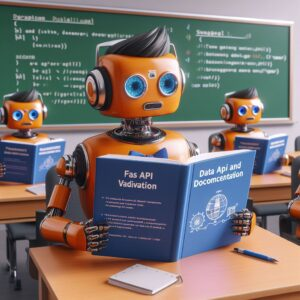
Embracing Asynchronous Programming
FastAPI is built on ASGI, making asynchronous programming a first-class citizen. This enables better performance under heavy loads and when waiting on I/O operations.
Suppose you want to fetch user information from a (mock) async database call:
from fastapi import FastAPI
import asyncio
app = FastAPI()
async def fake_db_call(user_id: int):
await asyncio.sleep(1) # Simulate I/O-bound operation
return {"user_id": user_id, "username": f"user{user_id}"}
@app.get("/users/{user_id}")
async def read_user(user_id: int):
data = await fake_db_call(user_id)
return data
- Asynchronous Endpoints: Simply define your path function with
async def
and useawait
for async calls. - Concurrency: This allows the server to handle multiple requests concurrently, improving throughput and responsiveness.
Essential Tips and Best Practices
To maximize productivity and maintainability, keep these best practices in mind when developing with FastAPI:
- Use Dependency Injection: FastAPI’s dependency injection system helps manage authentication, database connections, and reusable components.
- Organize Your Project: Separate your routers, models, and config for scalability.
- Leverage Type Hints: Fully utilize type hints for automatic docs, validation, and editor support.
- Test Your API: Use tools like
pytest
and HTTPX for effective API testing. - Consider Production Deployment: Use servers like Gunicorn with Uvicorn workers (
gunicorn -k uvicorn.workers.UvicornWorker
) for robust deployments.
Conclusion
FastAPI makes building high-performance APIs in Python both approachable and enjoyable. Its modern features, blazing speed, and developer-friendly design help you move from prototype to production in record time. As you experiment further—adding authentication, background tasks, and database integrations—you’ll discover FastAPI’s true power and versatility.
Ready to Build Your Next API?
Jumpstart your next project with FastAPI today, and unlock the speed and simplicity that modern Python developers love. Happy coding!