- Published on
Demystifying Cloud Native Application Development Principles, Architecture, and Practical Implementation
- Authors
- Name
- Adil ABBADI
Introduction
Cloud native application development is rapidly transforming the software landscape, enabling organizations to build scalable, resilient, and flexible systems that harness the power of the cloud. By leveraging modern methodologies such as microservices, containers, and DevOps automation, cloud native empowers teams to deliver software faster and more reliably.
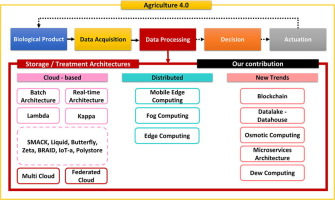
- What is Cloud Native Application Development?
- Building Blocks: Microservices, Containers, and Orchestration
- DevOps and Automation in the Cloud Native Ecosystem
- Best Practices for Cloud Native Success
- Conclusion
- Start Your Cloud Native Journey Today
What is Cloud Native Application Development?
At its core, cloud native application development is an approach to building and running apps that fully exploit the advantages of the cloud computing delivery model. This means designing software as a collection of loosely coupled, small, and independently deployable services (microservices) that run in containers and are orchestrated by platforms like Kubernetes.
Key tenets include:
- Microservices architecture: Decomposing apps into modular services.
- Containerization: Packaging applications and dependencies in containers.
- Dynamic orchestration: Using platforms like Kubernetes to manage service deployment and scaling.
- DevOps automation: Implementing CI/CD pipelines to streamline code delivery.
- Infrastructure as code: Managing environment configuration programmatically.
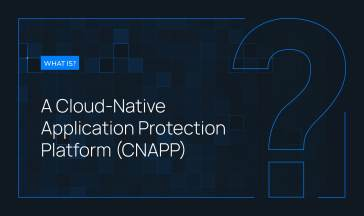
Building Blocks: Microservices, Containers, and Orchestration
Microservices in Practice
Microservices allow you to split applications into discrete components, each responsible for a specific functionality. For example, an e-commerce app might include user-service
, inventory-service
, and order-service
, communicating over APIs.
# Example: A lightweight Flask microservice
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/inventory')
def inventory():
return jsonify({'items': ['apple', 'banana', 'orange']})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
Benefits:
- Decoupled releases
- Technology diversity
- Fault isolation
Containerization with Docker
Containers encapsulate applications and their dependencies, ensuring a consistent environment across development, testing, and production. Docker is the de facto standard for containerization.
# Example Dockerfile for a Flask microservice
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Advantages:
- Portability
- Lightweight and fast startup
- Isolated environments
Orchestration with Kubernetes
As containerized applications scale, orchestration platforms like Kubernetes automate deployment, scaling, and management.
# Example Kubernetes Deployment for a microservice
apiVersion: apps/v1
kind: Deployment
metadata:
name: inventory-service
spec:
replicas: 3
selector:
matchLabels:
app: inventory
template:
metadata:
labels:
app: inventory
spec:
containers:
- name: inventory
image: username/inventory-service:latest
ports:
- containerPort: 5000
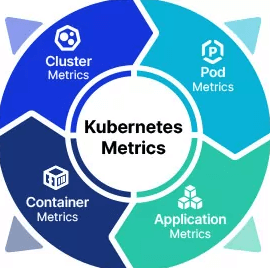
DevOps and Automation in the Cloud Native Ecosystem
Continuous Integration and Continuous Deployment (CI/CD) pipelines are critical in the cloud native lifecycle, enabling teams to quickly deliver changes with automated quality checks. Tools like GitHub Actions, GitLab CI, and Jenkins facilitate these workflows.
# Example GitHub Actions workflow for Docker image build and push
name: CI/CD Pipeline
on:
push:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Build Docker image
run: docker build -t username/inventory-service:latest .
- name: Push Docker image
run: docker push username/inventory-service:latest
Key DevOps practices:
- Automate testing and deployment pipelines
- Infrastructure as code (Terraform, Ansible)
- Robust monitoring and logging with Prometheus, Grafana, ELK stack
Best Practices for Cloud Native Success
- Design for failure: Build applications to gracefully handle failures and recover automatically.
- Leverage managed services: Offload operational overhead to cloud providers’ managed databases, queues, etc.
- Security first: Integrate security into your pipeline (DevSecOps), using techniques like image scanning and secrets management.
- Observability: Implement monitoring, logging, and tracing to gain real-time insights into app health and performance.
Conclusion
Cloud native application development enables organizations to innovate quickly, scale seamlessly, and adapt rapidly to change by combining microservices, containers, and modern DevOps practices. While the learning curve can be steep, the strategic benefits—agility, resilience, and speed—are well worth the investment.
Start Your Cloud Native Journey Today
Begin experimenting with microservices and containers, set up a simple Kubernetes cluster, or automate your first deployment pipeline. Embrace the cloud native mindset, and unlock new levels of software excellence!