- Published on
Exploring Microservices Architecture Patterns From Monoliths to Modular Ecosystems
- Authors
- Name
- Adil ABBADI
Introduction
Microservices architecture has transformed how we build robust, scalable, and maintainable systems. However, successfully adopting microservices isn't just about breaking up a monolith—it's about applying proven architectural patterns to manage complexity, enable independent development, and support rapid evolution. In this article, we’ll dive deep into the most pivotal microservices architecture patterns, illustrate their use with clear examples, and discuss when and how to leverage each for the best results.
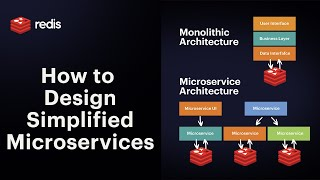
- The Decomposition Patterns: Breaking Up the Monolith
- Database Patterns: Managing Data in Microservices
- Communication Patterns: Reliable Service Interaction
- Conclusion
- Take the Next Step
The Decomposition Patterns: Breaking Up the Monolith
Decomposition is the beginning of the microservices journey. The goal is to identify suitable service boundaries that optimize deployment flexibility and codebase modularity. Two popular decomposition patterns are by business capability and by subdomain.
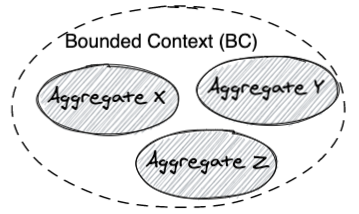
Decompose by Business Capability
Business capability decomposition divides services based on the core capabilities of an organization, often aligned with the team's responsibilities.
code // Example: E-commerce system decomposition
- Inventory Service
- Payment Service
- Shipping Service
Each service encapsulates its business logic and data, reducing coupling and enabling autonomous delivery pipelines.
Decompose by Subdomain
Leveraging domain-driven design (DDD), subdomain decomposition splits the system according to conceptual boundaries within the problem space.
code // Example: HR system decomposition
- Recruiting Subdomain Service
- Payroll Subdomain Service
- Onboarding Subdomain Service
This approach prevents the accidental creation of distributed monoliths and ensures clear ownership of business logic.
Database Patterns: Managing Data in Microservices
Microservices favor decentralized data management, but this introduces unique challenges. Three essential patterns are database per service, shared database, and the Saga pattern for transactions.
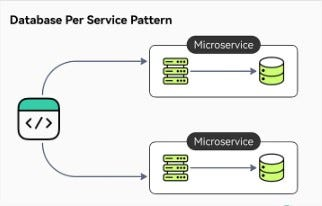
Database per Service
Each microservice manages its own database, eliminating tight coupling and aligning data models closely with service boundaries.
code // Example: Service-specific databases users-db (managed by User Service) orders-db (managed by Order Service)
However, queries spanning multiple services require specialized design patterns, such as API composition or CQRS.
Saga Pattern for Distributed Transactions
The Saga pattern coordinates distributed transactions via a series of local transactions, with each service triggering the next step through events.
code // Pseudocode for Saga pattern in a food ordering system Order Service: Create Order -> triggers Payment Service: Reserve Payment -> triggers Restaurant Service: Confirm Order -> triggers Delivery Service: Schedule Delivery
If any step fails, compensating actions are triggered, preserving eventual consistency across the system.
Communication Patterns: Reliable Service Interaction
Microservices must communicate effectively and reliably. Patterns like API Gateway, Choreography vs. Orchestration, and the Circuit Breaker address crucial communication needs.
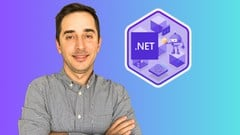
API Gateway Pattern
The API gateway acts as a single entry point for client requests, routing them to appropriate services, aggregating results, shielding internal details, and enforcing policies.
code // API Gateway routing configuration (conceptual) "/users/" routes to User Service "/orders/" routes to Order Service
This simplifies client logic while securing and shaping API traffic efficiently.
Choreography vs. Orchestration
- Choreography: Services communicate via events. Each service reacts independently, enabling loose coupling, but increasing complexity when observing workflows.
- Orchestration: A central orchestrator coordinates interactions, offering more control and observability.
code // Choreography (event-driven) Order Created Event -> Payment Service -> Payment Completed Event -> Shipping Service
// Orchestration (central controller) Orchestrator: Create Order -> Reserve Payment -> Schedule Shipping
Circuit Breaker Pattern
To safeguard against failures, the circuit breaker detects when a service is unavailable and prevents repeated, costly requests.
code if consecutiveFailures > threshold: openCircuit() // block downstream calls temporarily else: callService()
This improves system resilience and preserves resources during outages.
Conclusion
Microservices architecture is more than dividing your app into smaller pieces—it's about adopting patterns that address real-world scaling, reliability, and maintainability challenges. By mastering decomposition, data management, and communication patterns, teams can avoid common pitfalls and build systems ready for continuous evolution.
Take the Next Step
Experiment with these key patterns in your architecture. Explore case studies, try implementing small proof-of-concept services, and keep refining your boundary and integration strategies as you scale. Microservices success is a journey—make yours a well-patterned one!