- Published on
Unlocking the Power of WebAssembly Applications A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
WebAssembly (Wasm) is reshaping how we build web applications, delivering near-native performance, unlocking languages beyond JavaScript, and bridging the gap between web and native development. If you’ve ever wondered how to run complex applications – from games to image editors – directly inside your browser, WebAssembly is likely at play. In this article, we’ll dig deep into WebAssembly applications: what they are, where they're used, and how you can start building your own.
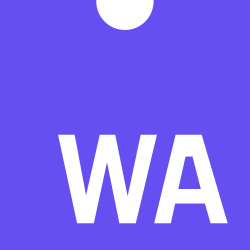
- What Is WebAssembly and Why It Matters
- Key Use Cases for WebAssembly Applications
- Integrating WebAssembly into a Modern Web App
- Real-World Examples and Performance Benchmarks
- Conclusion
- Ready to Dive In?
What Is WebAssembly and Why It Matters
WebAssembly is a binary instruction format designed to be executed at near-native speed by modern browsers. It works alongside JavaScript, opening the web to high-performance applications and languages like C, C++, Rust, and Go. The key advantage? It balances safety, portability, and speed – all within the security sandbox of the browser.
Consider this: before WebAssembly, JavaScript was the only viable language for web logic, and performance-intensive tasks (like video processing or 3D rendering) often struggled. Wasm changes the game, letting you build faster, richer web experiences and even reuse code from native projects.
Key Use Cases for WebAssembly Applications
WebAssembly isn’t just a theoretical improvement – it’s powering real-world tools today across multiple domains:
- Gaming and Graphics: AAA games (e.g., Unity WebGL builds) and advanced 3D editors
- Productivity Tools: Figma’s vector engine or AutoCAD in the browser
- Media Processing: In-browser video editors and transcoding
- Cross-Platform Apps: Porting legacy desktop software to the web
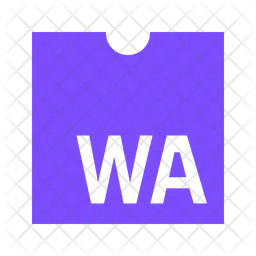
Let’s look at a concrete code example: compiling C to Wasm for a simple performance-heavy function.
// sum.c
int sum(int* arr, int length) {
int total = 0;
for (int i = 0; i < length; ++i) {
total += arr[i];
}
return total;
}
Compiled via Emscripten:
emcc sum.c -Os -s WASM=1 -o sum.js
Then, invoke from JavaScript:
Module.onRuntimeInitialized = () => {
var arrPtr = Module._malloc(4 * 10)
for (let i = 0; i < 10; i++) {
Module.HEAP32[(arrPtr >> 2) + i] = i
}
const result = Module._sum(arrPtr, 10)
console.log('Sum:', result)
Module._free(arrPtr)
}
Integrating WebAssembly into a Modern Web App
How do you bring Wasm into your own JavaScript-based project? The most common workflow involves compiling your source code (C/C++/Rust) to .wasm
, loading it in the browser, and then connecting with JavaScript APIs.
Here's a minimal Rust example:
// src/lib.rs
#[no_mangle]
pub fn add(a: i32, b: i32) -> i32 {
a + b
}
Compile with:
wasm-pack build --target web
In your JavaScript frontend:
import init, { add } from './pkg/mywasm.js'
async function run() {
await init()
console.log('Result:', add(2, 3))
}
run()
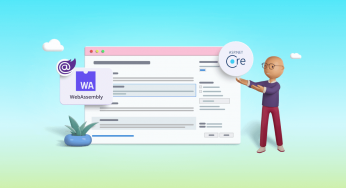
Real-World Examples and Performance Benchmarks
The shift to Wasm isn’t hypothetical – leading apps are already deploying it for major performance wins or simpler code reuse. For instance, Google Earth’s browser version leverages Wasm to process 3D terrain and imagery. AutoCAD ported millions of lines of C++ to run natively in browsers, drastically shortening load times and making heavy computational workloads feasible online.
A JS vs. Wasm performance benchmark (summing a large array):
// Pure JavaScript version
function sum(arr) {
let total = 0
for (const val of arr) total += val
return total
}
Versus the C/Wasm version above, which can be 2–10x faster for large datasets.
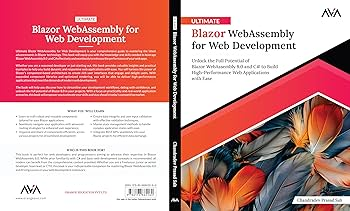
Performance gains will of course depend on the type of computation, but in scenarios involving loops, image manipulation, or math-heavy logic, Wasm is a clear winner.
Conclusion
WebAssembly is unlocking a powerful new era for web applications, bringing together safety, speed, and cross-platform compatibility. With expanding language support and robust tooling, it’s easier than ever to convert native code for web deployment or supercharge existing JavaScript projects. Now is the perfect time to explore what Wasm can do for your next app.
Ready to Dive In?
Start experimenting with WebAssembly by porting a small C or Rust function into your web project—discover the performance and versatility your apps can achieve! The web just got a lot faster—now, it’s your turn to build the future.