- Published on
Blockchain Development Fundamentals A Complete Guide for Beginners
- Authors
- Name
- Adil ABBADI
Introduction
Blockchain technology has rapidly transformed the world of software development by introducing decentralized architectures, trustless transactions, and automated smart contracts. Whether you’re a curious beginner or an experienced developer shifting into blockchain, understanding the essentials provides the foundation needed to create secure, decentralized applications.
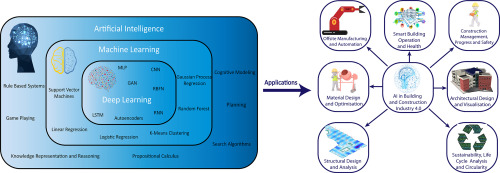
- Understanding Blockchain Architecture
- Smart Contracts: The Heart of Blockchain Logic
- Building Decentralized Applications (dApps)
- Conclusion
- Start Your Blockchain Journey
Understanding Blockchain Architecture
At its core, a blockchain is a distributed, immutable ledger that records transactions in sequential blocks. Each block is linked cryptographically to the previous one, forming a secure and transparent chain.
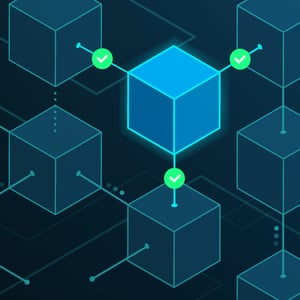
Key elements include:
- Nodes: Computers on the network storing a copy of the ledger.
- Blocks: Data containers storing transactions.
- Consensus Mechanisms: Protocols (like Proof of Work or Proof of Stake) used to validate new blocks.
Here’s a basic pseudocode representation of how a block is added:
class Block:
def __init__(self, previous_hash, data):
self.timestamp = get_current_time()
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def add_block(blockchain, data):
last_block = blockchain[-1]
new_block = Block(last_block.hash, data)
blockchain.append(new_block)
Smart Contracts: The Heart of Blockchain Logic
Smart contracts are self-executing agreements coded on the blockchain. They automatically enforce predefined rules, removing the need for intermediaries.
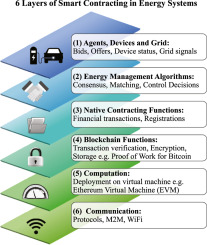
A typical smart contract on Ethereum (using Solidity) might look like this:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStore {
uint public storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
After compiling and deploying the contract with tools like Remix IDE, users interact directly with these functions, transparently recorded on the blockchain.
Building Decentralized Applications (dApps)
Decentralized applications (dApps) leverage blockchain backends, enabling trustless peer-to-peer interactions. A dApp typically consists of a smart contract, a web front end, and wallet integration for blockchain transactions.
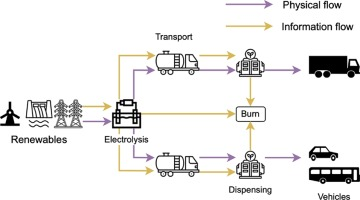
A simple web3.js example connects a web page to Ethereum:
// HTML: <button id="getData">Get Data</button>
if (window.ethereum) {
const web3 = new Web3(window.ethereum);
const contract = new web3.eth.Contract(contractABI, contractAddress);
document.getElementById('getData').onclick = async () => {
const data = await contract.methods.get().call();
alert('Stored Data: ' + data);
};
} else {
alert('No Ethereum wallet detected!');
}
Here, the dApp connects the interface to the blockchain, letting users read smart contract data with just a click.
Conclusion
Mastering the essentials of blockchain development—understanding its architecture, programming smart contracts, and building dApps—lays the groundwork for innovation in decentralized technology. With these core concepts and hands-on code samples, you’re prepared to dive deeper into the rapidly evolving blockchain ecosystem.
Start Your Blockchain Journey
Ready to build your first decentralized solution? Explore further, experiment with code, and join the expanding world of blockchain development!