- Published on
Building Robust GraphQL APIs A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
In the ever-evolving landscape of web development, GraphQL has emerged as a powerful alternative to REST for building flexible and efficient APIs. Unlike traditional REST endpoints, GraphQL empowers clients to request exactly the data they need, reducing over-fetching and under-fetching problems while increasing performance and developer productivity. Whether you’re an API novice or an experienced backend engineer, mastering GraphQL can vastly improve your ability to deliver modern, scalable applications.
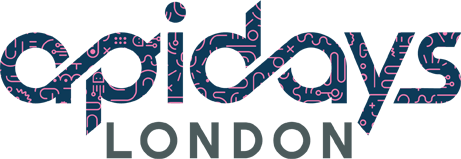
- Understanding the GraphQL Schema
- Resolvers: Powering Your Schema
- Mutations, Input Types, and Best Practices
- Conclusion
- Next Steps: Build and Experiment
Understanding the GraphQL Schema
A GraphQL API is driven by its schema — a strongly-typed system that defines how clients can access data. The schema specifies objects, their fields, relationships, and the types of queries and mutations available.
Defining a schema establishes a contract between the client and server, ensuring precise communication.
# schema.graphql
type User {
id: ID!
name: String!
email: String!
posts: [Post!]!
}
type Post {
id: ID!
title: String!
content: String!
author: User!
}
type Query {
allUsers: [User!]!
user(id: ID!): User
}
With this structure, clients can request users, their posts, or specific details as needed.
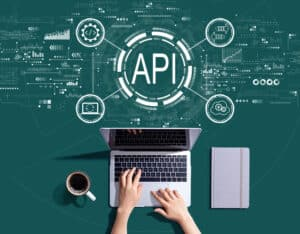
Resolvers: Powering Your Schema
Schema fields need logic to fetch or modify data—this is where resolvers come in. A resolver is a function that returns data for a field in your schema, allowing you to connect your schema to any kind of backend or database.
For a Node.js server using Apollo Server, resolvers might be set up as follows:
// resolvers.js
const resolvers = {
Query: {
allUsers: async (_, __, { dataSources }) => dataSources.usersAPI.getAllUsers(),
user: async (_, { id }, { dataSources }) => dataSources.usersAPI.getUserById(id),
},
User: {
posts: (user, _, { dataSources }) => dataSources.postsAPI.getPostsByUser(user.id),
},
Post: {
author: (post, _, { dataSources }) => dataSources.usersAPI.getUserById(post.authorId),
},
};
Resolvers provide the link between your API and your data, enabling advanced features like authorization, batching, and caching.
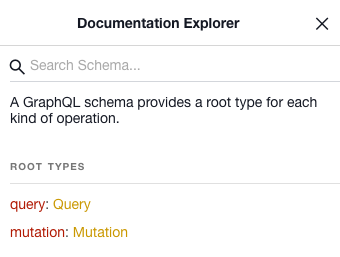
Mutations, Input Types, and Best Practices
Beyond queries, GraphQL’s mutation system allows you to modify server-side data, such as creating, updating, or deleting records. Mutations typically take input types for structured arguments.
Here’s an example mutation definition and its resolver:
# schema.graphql
input CreatePostInput {
title: String!
content: String!
authorId: ID!
}
type Mutation {
createPost(input: CreatePostInput!): Post!
}
// resolvers.js
const resolvers = {
Mutation: {
createPost: async (_, { input }, { dataSources }) =>
dataSources.postsAPI.createPost(input),
},
};
Best Practices:
- Type safety: Use input types to standardize arguments.
- Error handling: Always provide meaningful errors and responses.
- Validation: Validate and sanitize input at the resolver level.
Conclusion
GraphQL API development modernizes data fetching, making APIs more expressive and efficient. With a well-designed schema, robust resolvers, and thoughtful use of queries and mutations, you can build backends that delight frontend developers and users alike. By following best practices, you ensure maintainability and scalability for your growing applications.
Next Steps: Build and Experiment
Ready to level up? Start building your own GraphQL server, experiment with advanced concepts like subscriptions, batching, and custom directives, and explore cloud-hosted GraphQL solutions for production-grade APIs. Happy coding!