- Published on
Building Scalable Systems with Node.js Microservices Development
- Authors
- Name
- Adil ABBADI
Introduction
In today’s fast-paced software landscape, scalability, maintainability, and rapid deployment are paramount. Node.js microservices architecture empowers developers to build modular, independently deployable services that handle specific business capabilities, resulting in robust and agile systems. This article will delve into the principles and practice of Node.js microservices development with concrete code snippets and actionable insights.
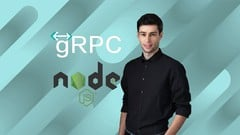
- Why Choose Microservices With Node.js?
- Designing Node.js Microservices: Core Concepts
- Implementing a Simple Node.js Microservice System
- Best Practices for Node.js Microservices
- Conclusion
- Ready to Build Your Node.js Microservices System?
Why Choose Microservices With Node.js?
Monolithic applications quickly become a bottleneck as projects scale, slowing down development and deployment cycles. Microservices split a large system into small, isolated services, each responsible for a distinct task, making them easier to develop, test, and scale independently.
Node.js is particularly suited for microservices because of:
- Event-driven, non-blocking I/O: Handles many connections efficiently.
- Lightweight footprint: Low memory usage makes it ideal for microservices.
- Extensive ecosystem: Rich npm modules streamline implementation.
- Rapid development: JavaScript familiarity across full-stack teams.
Designing Node.js Microservices: Core Concepts
Successful microservices depend on thoughtful architectural design:
1. Defining Service Boundaries
Decide what business functionality each microservice will encapsulate. Clear boundaries reduce coupling and ease deployment.
2. Communication Between Services
Common patterns include REST APIs, gRPC, and message queues (like RabbitMQ or Kafka).
// Example: Express-based product microservice in Node.js
const express = require('express')
const app = express()
app.use(express.json())
app.get('/products', (req, res) => {
res.json([
{ id: 1, name: 'Laptop' },
{ id: 2, name: 'Phone' },
])
})
app.listen(4000, () => console.log('Product service running on port 4000'))
3. Service Discovery and Load Balancing
Use service registries (like Consul or etcd) and load balancers to direct traffic and maintain resilience as services scale dynamically.
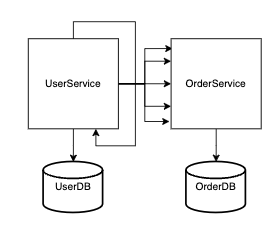
Implementing a Simple Node.js Microservice System
Let’s walk through building two microservices: one for managing users, and another for handling orders.
1. The User Service
Handles user registration and retrieval.
// services/user-service.js
const express = require('express')
const app = express()
let users = [{ id: 1, name: 'Alice' }]
app.use(express.json())
app.post('/users', (req, res) => {
const user = { id: users.length + 1, ...req.body }
users.push(user)
res.status(201).json(user)
})
app.get('/users', (req, res) => {
res.json(users)
})
app.listen(5000, () => console.log('User service running on port 5000'))
2. The Order Service
Stores orders and needs to communicate with the User Service.
// services/order-service.js
const express = require('express')
const axios = require('axios')
const app = express()
let orders = []
app.use(express.json())
app.post('/orders', async (req, res) => {
// Validate user exists
const { userId, item } = req.body
const { data: users } = await axios.get('http://localhost:5000/users')
if (!users.some((u) => u.id === userId)) {
return res.status(400).json({ error: 'User not found.' })
}
const order = { id: orders.length + 1, userId, item }
orders.push(order)
res.status(201).json(order)
})
app.get('/orders', (req, res) => res.json(orders))
app.listen(5001, () => console.log('Order service running on port 5001'))
3. API Gateway Pattern
Centralize incoming requests and route them to appropriate services, handling concerns like authentication and rate limiting.
// gateway/api-gateway.js
const express = require('express')
const proxy = require('http-proxy-middleware').createProxyMiddleware
const app = express()
app.use('/users', proxy({ target: 'http://localhost:5000', changeOrigin: true }))
app.use('/orders', proxy({ target: 'http://localhost:5001', changeOrigin: true }))
app.listen(8080, () => console.log('API Gateway running on port 8080'))
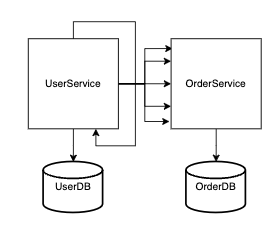
Best Practices for Node.js Microservices
Building production-grade Node.js microservices involves more than splitting code:
- Error Handling: Implement centralized error logging (e.g., Winston, ELK).
- Monitoring & Health Checks: Use tools like Prometheus, Grafana, and expose
/health
endpoints. - Data Management: Favor decentralized databases, but plan for data consistency and transactions if necessary.
- Testing: Isolate services and use contract testing (e.g., Pact) when APIs change.
- Security: Secure inter-service communication with HTTPS and JWT.
Conclusion
With microservices, Node.js brings unmatched speed and flexibility to constructing scalable distributed systems. Adopting this architecture enables autonomous teams, easier scaling, and resilience, but also requires intentional design, robust communication patterns, and smart tooling.
Ready to Build Your Node.js Microservices System?
Get started by defining clear service boundaries and experimenting with a basic setup; scale your implementation as your confidence grows. Dive deeper into the ecosystem, enhance automation, and join the thriving community to unlock the full potential of microservices with Node.js!