- Published on
Mastering Jenkins Pipeline Automation Best Practices and Workflows
- Authors
- Name
- Adil ABBADI
Introduction
In the ever-evolving landscape of software development, automation is at the heart of fast, reliable delivery pipelines. Jenkins, as a leading open source automation server, empowers teams with powerful pipeline automation tools that orchestrate complex CI/CD processes with ease. This article dives deep into Jenkins Pipeline automation, offering best practices, practical pipelines, and operational insights to supercharge your development cycle.
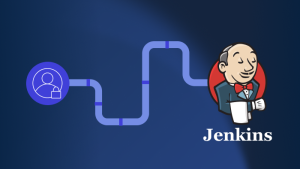
- Understanding Jenkins Pipelines
- Automating Builds and Tests
- Continuous Deployment and Notifications
- Conclusion
- Get Started with Jenkins Pipeline Automation!
Understanding Jenkins Pipelines
Jenkins Pipelines offer a robust way to define, visualize, and manage complex build and deployment flows as code. Pipelines are created using a simple, expressive DSL (Declarative or Scripted) that lives in your source repository, ensuring versioned and reviewable automation.
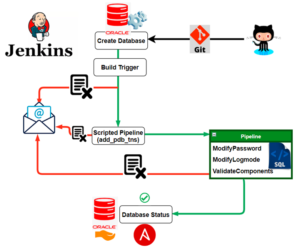
By defining your build, test, and deployment process in a Jenkinsfile, you gain repeatability, scalability, and visibility into your automation. There are two main types of Jenkins Pipelines:
- Declarative Pipeline: User-friendly with a straightforward syntax.
- Scripted Pipeline: More flexible, using Groovy for advanced flows.
// Basic Declarative Pipeline Example
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building the project...'
}
}
stage('Test') {
steps {
echo 'Running tests...'
}
}
stage('Deploy') {
steps {
echo 'Deploying application...'
}
}
}
}
Automating Builds and Tests
Automating the build and test process ensures that every code push gets validated, reducing manual work and errors. Jenkins Pipelines allow seamless integration with build tools and test frameworks:
- Integrating with Maven or Gradle: Automatically compile and test Java projects.
- Automating with Docker: Build, test, and publish Docker images.
- Parallel Testing: Speed up feedback by running tests concurrently.
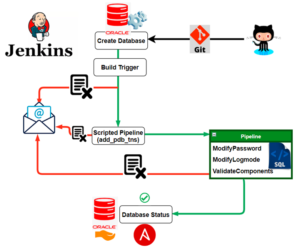
pipeline {
agent any
environment {
DOCKER_IMAGE = 'my-app:${BUILD_NUMBER}'
}
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Docker Build & Test') {
steps {
sh 'docker build -t $DOCKER_IMAGE .'
sh 'docker run --rm $DOCKER_IMAGE test'
}
}
stage('Publish') {
steps {
sh 'docker push $DOCKER_IMAGE'
}
}
}
}
Automated builds and tests provide a foundation for quality and confidence in software delivery.
Continuous Deployment and Notifications
Jenkins Pipelines streamline deployment to any environment—development, staging, or production. You can integrate with tools like Kubernetes, Ansible, AWS, or on-prem servers, and keep stakeholders informed through notifications.
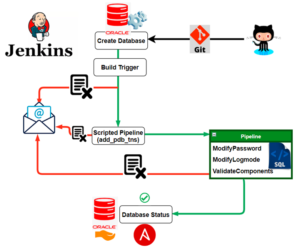
- Controlled Deployments: Use input steps for manual approvals before deploying to production.
- Slack/Email Notifications: Send alerts when jobs fail or succeed.
pipeline {
agent any
stages {
stage('Deploy to Staging') {
steps {
sh 'ansible-playbook deploy.yml -e env=staging'
}
}
stage('Approval') {
steps {
input('Deploy to production?')
}
}
stage('Deploy to Production') {
steps {
sh 'ansible-playbook deploy.yml -e env=production'
}
}
}
post {
failure {
mail to: 'dev-team@example.com',
subject: "Pipeline Failed: ${env.JOB_NAME}",
body: "The Jenkins pipeline has failed.\nSee details at: ${env.BUILD_URL}"
}
success {
slackSend channel: '#deployments', message: "Deployment succeeded for ${env.JOB_NAME}"
}
}
}
Automated deployments with notifications bring transparency, traceability, and control to your CI/CD process.
Conclusion
Jenkins Pipeline automation revolutionizes how teams approach continuous integration and delivery. By defining processes as code, leveraging automated testing, and orchestrating seamless deployments, organizations can achieve faster, safer, and more reliable software delivery. Mastering Jenkins Pipelines is key to thriving in today’s DevOps-driven world.
Get Started with Jenkins Pipeline Automation!
Embrace Jenkins Pipeline automation today—explore, experiment, and elevate your development workflows for increased productivity and quality. Want to learn more? Dive into the Jenkins Pipeline documentation and start building smarter CI/CD pipelines!