- Published on
Mastering Model-Context-Pattern Implementation for Scalable Software Design
- Authors
- Name
- Adil ABBADI
Introduction
As software systems grow in complexity, it becomes increasingly challenging to manage the intricate relationships between components, leading to rigidity, fragility, and maintainability issues. To overcome these hurdles, software architects must adopt design patterns that promote modularity, scalability, and reusability. One such pattern is the Model-Context-Pattern implementation, which has gained popularity in recent years due to its ability to separate concerns and enable flexible architecture. This comprehensive guide will delve into the inner workings of the Model-Context-Pattern implementation, explaining its core components, benefits, and practical implementation strategies.
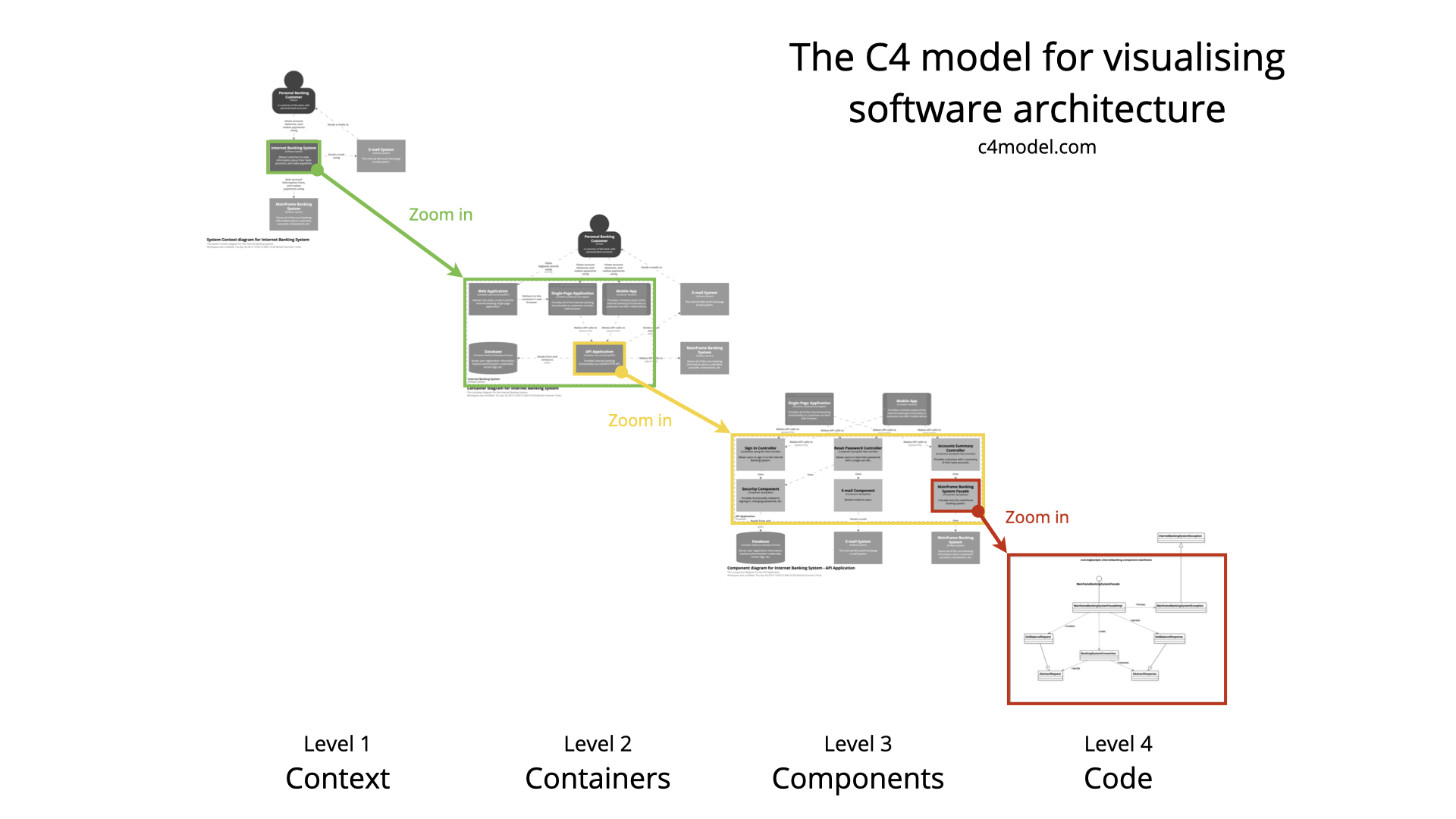
Defining the Model
The Model represents the core business logic of the application, encapsulating the rules, data, and behavior of the domain. In the Model-Context-Pattern implementation, the Model serves as the single source of truth, providing a unified view of the business logic. This enables the development of a robust, modular, and maintainable software system.
// Example of a simple User class in Java
public class User {
private String id;
private String name;
private String email;
public User(String id, String name, String email) {
this.id = id;
this.name = name;
this.email = email;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
Understanding the Context
The Context represents the environment in which the Model with the necessary information to operate. It acts as an adaptor, providing the Model with access to external dependencies, such as databases, APIs, or file systems. By decoupling the Model from external dependencies, the Context enables the development of a flexible architecture, allowing for easy substitution of dependencies without affecting the Model.
// Example of a Context class in Kotlin
class UserContext(private val userRepository: UserRepository) {
fun getUser(id: String): User {
val user = userRepository.findUserById(id)
return User(user.id, user.name, user.email)
}
}
Implementing the Pattern
The Pattern represents the bridge between the Model and the Context, defining how the Model interacts with the Context. This enables the Model to request data or perform actions without being aware of the underlying Context.
// Example of a simple Pattern class in Java
public interface UserPattern {
User getUser(String id);
}
public class UserRepositoryPattern implements UserPattern {
private final UserContext userContext;
public UserRepositoryPattern(UserContext userContext) {
this.userContext = userContext;
}
public User getUser(String id) {
return userContext.getUser(id);
}
}
Benefits and Advantages
The Model-Context-Pattern implementation offers numerous benefits, including:
- Separation of Concerns: By separating the business logic from the infrastructure and dependencies, the Model-Context-Pattern implementation enables the development of a modular architecture.
- Scalability: The decoupling of the Model from external dependencies, allows for easy substitution of dependencies, enabling scalability and flexibility.
- Reusability: The modular nature of the pattern enables the reuse of components across different applications and domains.
Conclusion
In conclusion, the Model-Context-Pattern implementation is a powerful design pattern that enables the development of scalable, maintainable software systems. By separating concerns, promoting modularity, and enabling flexibility, this pattern has become a cornerstone of modern software architecture. As software architects, it is essential to master this pattern, unlocking the full potential of your software systems.
Take Your Design to the Next Level
Mastering the Model-Context-Pattern implementation is just the beginning. Stay ahead of the curve by exploring other cutting-edge design patterns, such as Domain-Driven Design and Microservices Architecture.