- Published on
Rust Programming for Systems A Modern Approach to Systems Development
- Authors
- Name
- Adil ABBADI
Introduction
Systems programming—the low-level creation of operating systems, file systems, embedded code, and high-performance network servers—has long been associated with C and C++. However, the Rust programming language is rapidly gaining ground as a safer, more ergonomic alternative, combining powerful abstractions with uncompromising performance and reliability. In this article, we'll explore how Rust is revolutionizing systems programming, illustrating key concepts with practical examples.
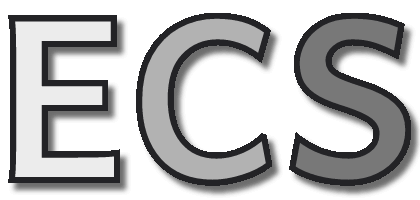
- Why Rust for Systems Programming?
- The Rust Ownership Model in Practice
- System-Level Performance: Building a Minimal Web Server
- Conclusion
- Ready to Unlock the Power of Rust in Your Systems Code?
Why Rust for Systems Programming?
Rust was explicitly designed to tackle the complexities of systems-level work. From operating system kernels to device drivers and network services, Rust rewrites the rules with its blend of memory safety, thread safety, and zero-cost abstractions.
- Memory Safety without Garbage Collection: Rust’s ownership model eliminates entire classes of bugs like dangling pointers and data races.
- Performance: Rust's abstractions compile away, resulting in performance on par with C/C++.
- Concurrency: Fearless concurrency is possible due to strict compile-time guarantees.
fn main() {
let data = vec![1, 2, 3, 4, 5];
let handle = std::thread::spawn(move || {
println!("Thread data: {:?}", data);
});
handle.join().unwrap();
}
This example shows how Rust makes concurrent programming easy and safe, as ownership moves into the thread, guaranteeing there are no data races.
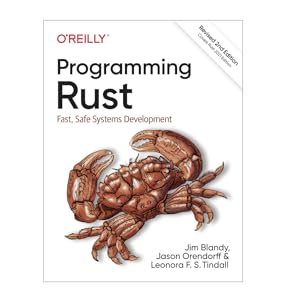
The Rust Ownership Model in Practice
One of Rust’s defining features is its “ownership system,” which ensures safe and predictable memory usage—paramount in systems code.
- Borrowing and Lifetimes: Ownership enables memory safety without a garbage collector by rigorously tracking variable scopes and references.
- Zero-cost Abstractions: Rust's rules are checked at compile-time, introducing no runtime overhead.
fn print_length(vec: &Vec<i32>) {
println!("Vector length: {}", vec.len());
}
fn main() {
let v = vec![10, 20, 30];
print_length(&v); // pass by reference safely
println!("Still valid: {:?}", v); // v can still be used
}
By borrowing instead of taking ownership, the print_length
function guarantees safe, concurrent access with no need for manual memory management.
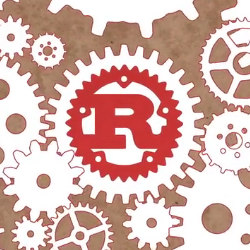
System-Level Performance: Building a Minimal Web Server
To demonstrate Rust’s power, let’s implement a tiny single-threaded HTTP server using the standard library. This shows how close-to-the-metal efficiency and modern language features join force.
use std::io::prelude::*;
use std::net::TcpListener;
use std::net::TcpStream;
fn handle_client(mut stream: TcpStream) {
let mut buffer = [0; 512];
stream.read(&mut buffer).unwrap();
let response = "HTTP/1.1 200 OK\r\n\r\nHello, world!";
stream.write(response.as_bytes()).unwrap();
stream.flush().unwrap();
}
fn main() {
let listener = TcpListener::bind("127.0.0.1:7878").unwrap();
for stream in listener.incoming() {
handle_client(stream.unwrap());
}
}
This minimalist web server demonstrates how straightforward and safe systems programming can be with Rust—without sacrificing performance.
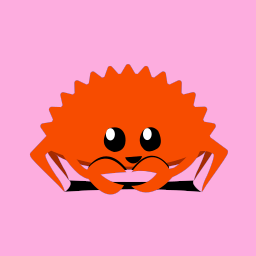
Conclusion
Rust brings powerful promises to the realm of systems programming: uncompromising safety, modern ergonomics, and the high performance demanded by low-level work. Whether you’re building kernel modules, network applications, or embedded firmware, Rust provides the tools to do so reliably and efficiently.
Ready to Unlock the Power of Rust in Your Systems Code?
Dive into the Rust Book, join the thriving community, and try porting a small systems project to Rust—you'll discover a whole new way to program close to the hardware with confidence!