- Published on
MongoDB Database Optimization Best Practices for High Performance
- Authors
- Name
- Adil ABBADI
Introduction
Optimizing your MongoDB database is crucial for handling large-scale applications, ensuring low latency, and maintaining high throughput. Whether you’re running a startup MVP or a distributed enterprise app, a well-tuned MongoDB instance can make the difference between a snappy user experience and frequent slowdowns. In this article, we’ll explore practical optimization tactics with clear explanations, actionable code examples, and visual guides to help you achieve peak MongoDB performance.
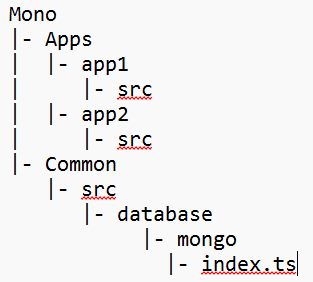
- Schema Design Strategies
- Indexing for Fast Queries
- Query Optimization Techniques
- Scaling and Resource Management
- Conclusion
- Take Your MongoDB to the Next Level
Schema Design Strategies
Successful MongoDB optimization starts at the schema level. By designing collections and documents to align with your querying patterns and data access needs, you’ll avoid many common performance headaches.
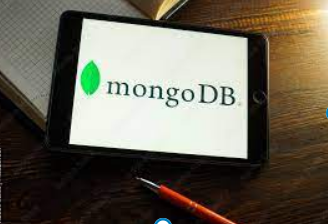
MongoDB is schema-less, but enforcing structure through clear conventions yields tangible benefits for performance. Consider embedding versus referencing:
// Embedding example: Store comments directly within post document
{
_id: ObjectId("..."),
title: "Effective MongoDB Schema",
comments: [
{ user: "alice", text: "Great tips!" },
{ user: "bob", text: "Very helpful." }
]
}
// Referencing example: Maintain related collections and use $lookup or manual joins
{
_id: ObjectId("postId"),
title: "Effective MongoDB Schema"
}
{
_id: ObjectId("commentId"),
postId: ObjectId("postId"),
user: "alice",
text: "Great tips!"
}
Tips:
- Embed for “contains” relationships and frequently-read subdocuments.
- Reference for large, infrequently-accessed, or many-to-many related data.
Indexing for Fast Queries
Indexes are central to making your MongoDB queries performant. Without indexes, MongoDB resorts to full collection scans—fine for small datasets, disastrous for production volumes.
Create compound, single-field, or TTL indexes to match your query workloads:
// Create a compound index on "user_id" and "created_at"
db.orders.createIndex({ user_id: 1, created_at: -1 })
// TTL Index for expiring documents (such as sessions)
db.sessions.createIndex({ lastAccess: 1 }, { expireAfterSeconds: 3600 })
// Unique indexes to prevent duplicate entries
db.users.createIndex({ email: 1 }, { unique: true })
Tips:
- Use
explain()
for queries to verify index usage. - Avoid too many indexes—they increase write costs.
- Regularly review and drop unused indexes.
Query Optimization Techniques
Efficient data retrieval depends not only on schema and indexes but also on how you structure your queries. MongoDB’s query planner can be influenced by your usage patterns.
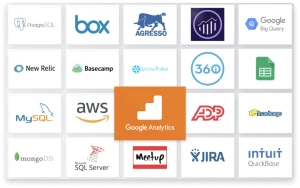
Consider these optimization tactics:
// Analyzing a query with explain()
db.orders.find({ user_id: "12345", status: "shipped" }).explain("executionStats")
// Projection: Only retrieve fields you need
db.users.find({ active: true }, { name: 1, email: 1 })
// Pagination with range queries (avoids skip/limit performance pitfall)
db.logs.find({ timestamp: { $gt: ISODate("2025-01-01") } })
.sort({ timestamp: 1 })
.limit(100)
Tips:
- Use projections to minimize data transfer.
- Prefer range-based pagination over
.skip()
, which can slow as collections grow. - Regularly monitor slow queries in the MongoDB logs.
Scaling and Resource Management
Optimizing at the database level is incomplete without considering scaling and resource levers: sharding, replication, and resource tuning.
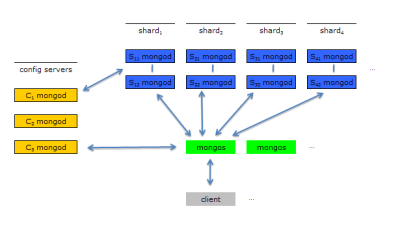
Tactics include:
// Enabling sharding for a database and collection
sh.enableSharding("myDatabase")
sh.shardCollection("myDatabase.users", { user_id: "hashed" })
// Adjusting WiredTiger cache size (in mongod.conf)
storage:
wiredTiger:
engineConfig:
cacheSizeGB: 4
Tips:
- Use sharding to scale out horizontally under high load.
- Tune WiredTiger cache size to fit read-mostly workload patterns.
- Ensure replication is configured for high availability.
Conclusion
MongoDB database optimization is a holistic, ongoing process involving mindful schema design, targeted indexing, query efficiency, and infrastructure scaling. By applying these best practices and regularly analyzing your database metrics, you can ensure your apps remain fast, reliable, and scalable—even as your data and user base grow.
Take Your MongoDB to the Next Level
Ready to supercharge your MongoDB performance? Start experimenting with these optimization strategies today—monitor, measure, and iterate for continuous improvement! For more hands-on tips, check out MongoDB’s robust documentation and community best practices.