- Published on
Terraform Infrastructure as Code Best Practices A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
Terraform has revolutionized the way teams provision and manage cloud infrastructure. By allowing developers to codify infrastructure as code (IaC), Terraform makes it easier to automate, version, scale, and reproduce entire environments with confidence. However, leveraging Terraform effectively requires adherence to best practices that ensure reliability, security, scalability, and team collaboration.
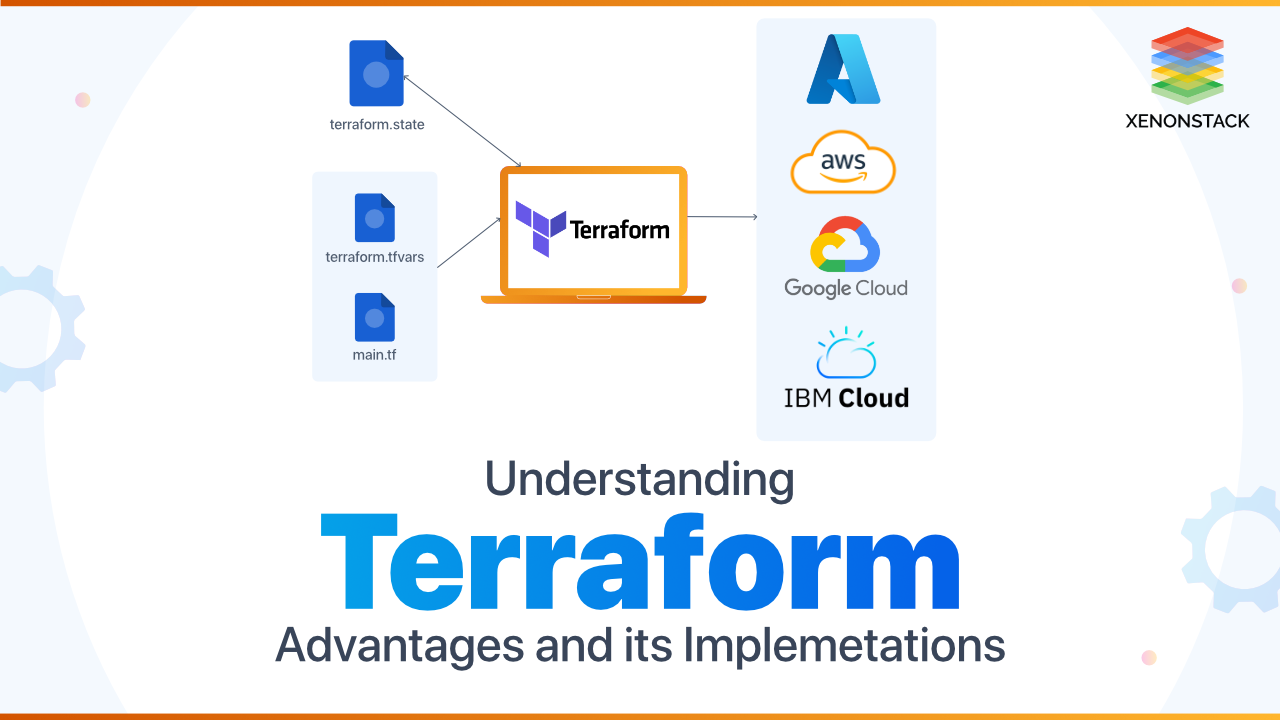
- Structuring Terraform Code for Maintainability
- Managing Terraform State Securely
- Version Control and Collaboration Strategies
- Conclusion
- Take Your Terraform to the Next Level
Structuring Terraform Code for Maintainability
Organizing your Terraform configuration is the foundation of scalable infrastructure. Proper structure enables ease of understanding, modularity, and agility in updates.
Use Modules to Encapsulate Components
Creating reusable modules helps isolate resources, minimize duplication, and standardize deployments.
# main.tf
module "network" {
source = "./modules/network"
vpc_cidr = "10.0.0.0/16"
}
module "compute" {
source = "./modules/compute"
instance_count = 3
subnet_id = module.network.subnet_id
}
Adopt a Logical Directory Layout
Organize configurations by environment and component for clarity:
.
├── modules/
│ └── network/
│ └── compute/
├── prod/
│ └── main.tf
├── staging/
│ └── main.tf
└── variables.tf
Separate State Files Per Environment
Separating state reduces blast radius and enhances environment isolation.
terraform {
backend "s3" {
bucket = "my-terraform-state"
key = "prod/terraform.tfstate"
region = "us-east-1"
}
}
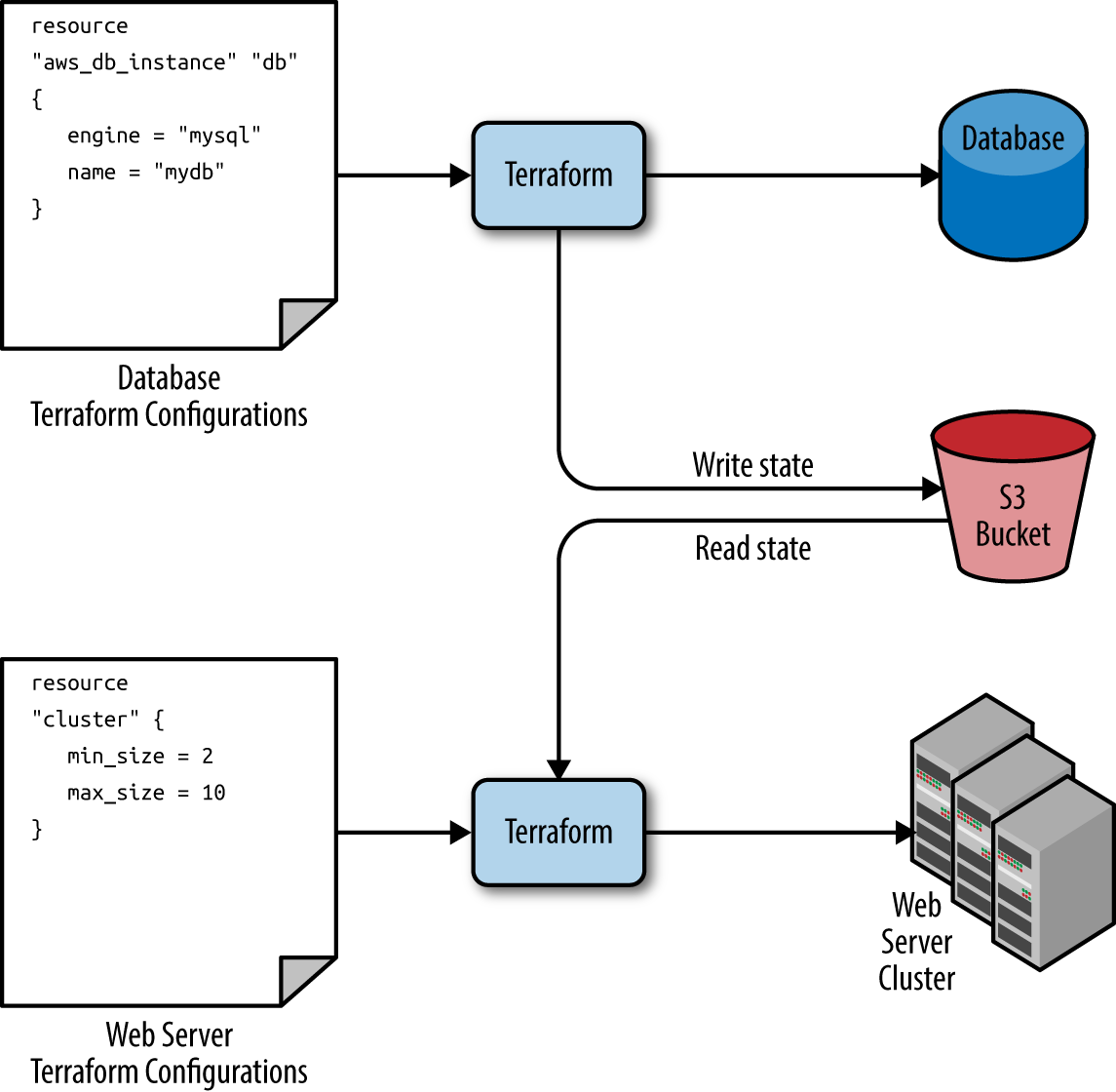
Managing Terraform State Securely
Mismanaged Terraform state can lead to data leaks or lost infrastructure tracking. Adopt these practices:
Store State Remotely and Securely
Leverage backends like AWS S3 with state locking in DynamoDB.
terraform {
backend "s3" {
bucket = "iac-state"
key = "env/prod.tfstate"
region = "us-west-2"
dynamodb_table = "terraform-lock"
encrypt = true
}
}
Enable State Locking
State locking prevents concurrent modifications that could cause corruption.
- For S3: configure the DynamoDB lock table.
- For other clouds (GCP, Azure), use their locking mechanisms.
Never Commit State to Version Control
Always add .terraform/
and *.tfstate
to your .gitignore
.
# .gitignore
*.tfstate
*.tfstate.*
.terraform/
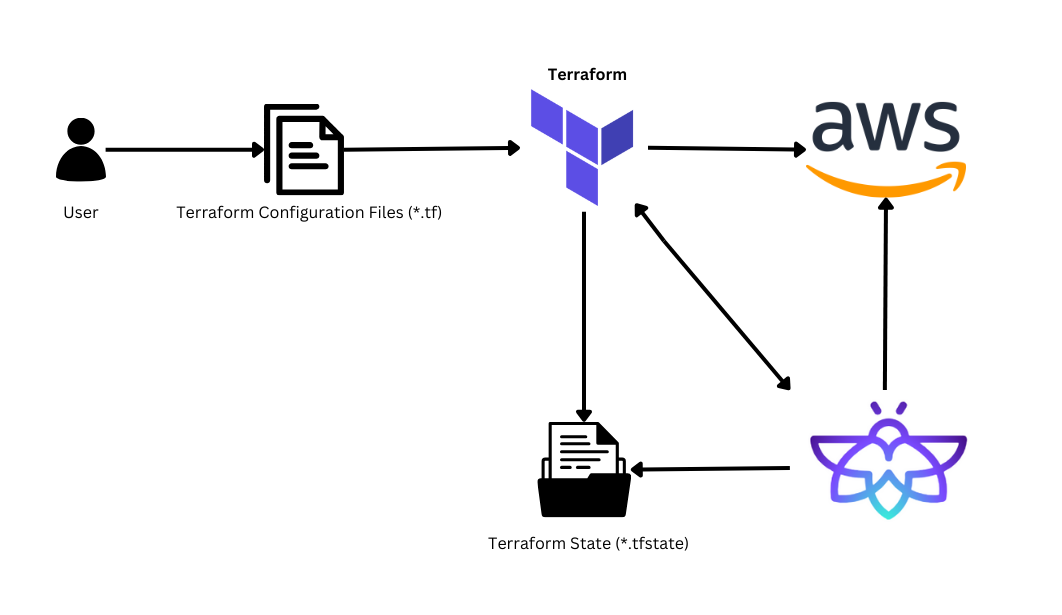
Version Control and Collaboration Strategies
Collaborative infrastructure management thrives on clear processes and automation.
Employ Code Reviews and Pull Requests
Treat IaC like application code: use pull/merge requests for all changes. This ensures peer review and early detection of issues.
Use Terraform Workspaces for Environments
Workspaces allow you to manage multiple environments from the same code base.
terraform workspace new dev
terraform workspace new prod
terraform workspace select prod
Integrate with CI/CD
Leverage Continuous Integration for plan and apply pipelines:
- Run
terraform plan
on PRs for preview. - Apply only after successful reviews and approvals.
A sample workflow might use GitHub Actions:
- name: Terraform Init
run: terraform init
- name: Terraform Plan
run: terraform plan -out=tfplan
- name: Terraform Apply
if: github.ref == 'refs/heads/main'
run: terraform apply -auto-approve tfplan
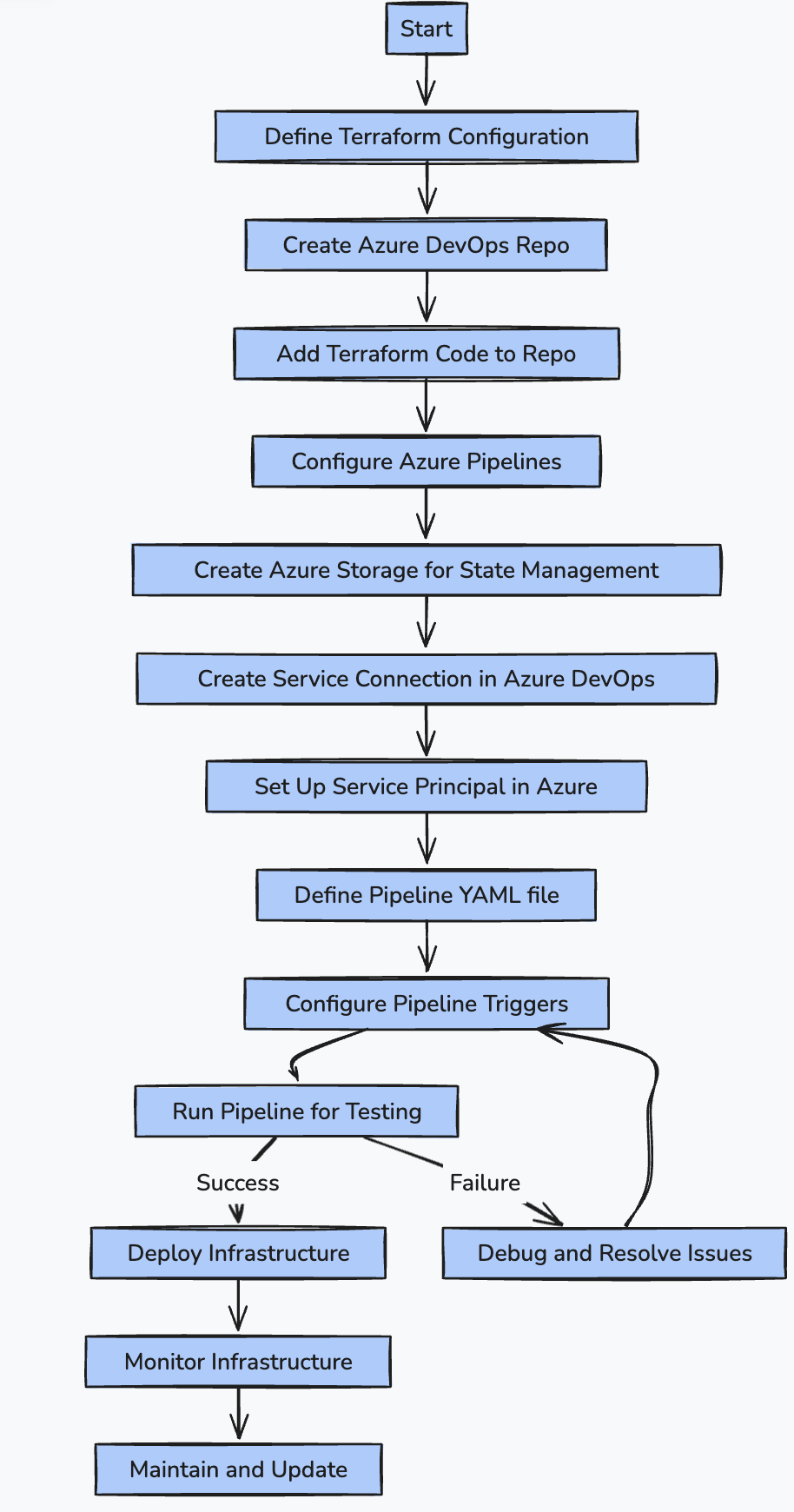
Conclusion
Terraform empowers teams to provision and manage infrastructure at scale, but true success comes from implementing best practices around code organization, state management, and collaboration. Following these guidelines ensures your IaC remains secure, maintainable, and ready for rapid innovation.
Take Your Terraform to the Next Level
Ready to improve your cloud infrastructure workflow? Start refactoring your codebases with these best practices, implement secure state storage, and automate your deployment pipeline. Explore more from the official Terraform docs and join the DevOps revolution!