- Published on
The Power and Potential of Machine Learning A Deep Dive
- Authors
- Name
- Adil ABBADI
Introduction
Machine learning (ML) is revolutionizing industries, powering everything from personalized recommendations on streaming platforms to self-driving cars. As an exciting subfield of artificial intelligence, machine learning enables systems to learn from data, identify patterns, and make decisions with minimal human intervention.
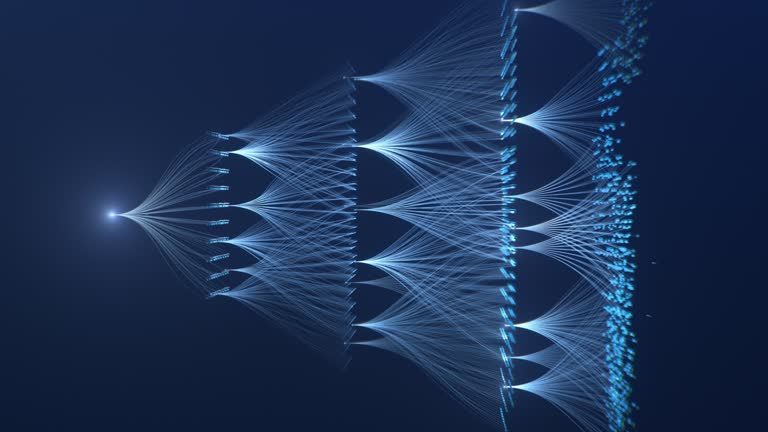
- What is Machine Learning?
- Essential Machine Learning Algorithms
- Real-World Applications of Machine Learning
- Conclusion
- Ready to Start Your ML Journey?
What is Machine Learning?
At its core, machine learning is the study of algorithms that improve automatically through experience. Instead of relying solely on explicit programming, ML systems learn from historical data and use that knowledge to make predictions or decisions.
Key concepts in machine learning:
- Data: The foundation of all learning, ranging from text to images and numbers.
- Models: Mathematical representations trained to detect patterns in data.
- Training: The process of teaching the model using known data.
The basic workflow for a typical ML model:
- Collect and prepare data.
- Choose and configure an algorithm.
- Train the model on the data.
- Evaluate the model’s performance.
- Deploy the model for real-world usage.
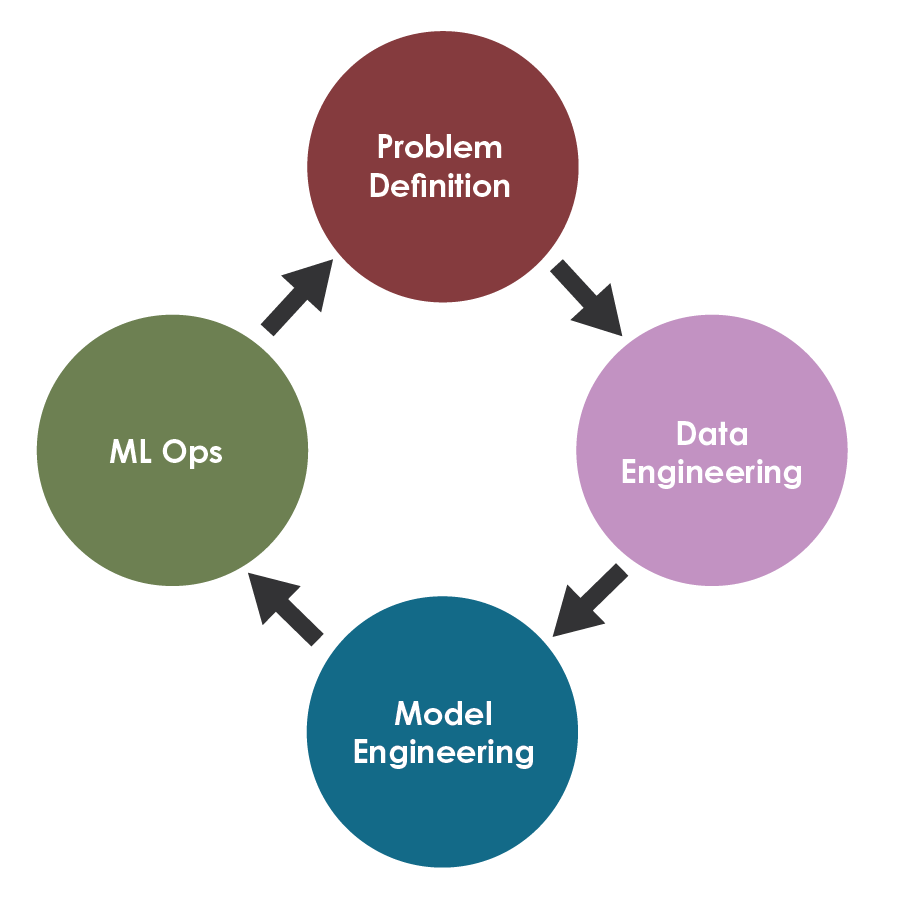
Essential Machine Learning Algorithms
Machine learning comprises a rich tapestry of algorithms, each suited for specific types of tasks. Broadly, these can be categorized as follows:
1. Supervised Learning
In supervised learning, the model is trained on labeled data — data paired with correct outputs. You might use this for classification (e.g., spam detection) or regression (e.g., price prediction).
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
# Load dataset
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(
iris.data, iris.target, test_size=0.2, random_state=42)
# Train logistic regression model
model = LogisticRegression(max_iter=200)
model.fit(X_train, y_train)
# Evaluate model
accuracy = model.score(X_test, y_test)
print(f"Test Accuracy: {accuracy:.2f}")
2. Unsupervised Learning
Unsupervised models explore data without explicit labels, discovering hidden patterns or groupings.
from sklearn.cluster import KMeans
import numpy as np
# Generate sample 2D data
X = np.random.rand(100, 2)
# Train KMeans clustering model
kmeans = KMeans(n_clusters=3, random_state=42)
kmeans.fit(X)
# Assign labels to clusters
labels = kmeans.labels_
print("Cluster labels assigned to data points:", labels[:10])
3. Reinforcement Learning
In reinforcement learning, an agent learns by interacting with an environment, receiving rewards or penalties for actions taken. Applications include robotics, game playing, and autonomous vehicles.
# Example pseudocode for Q-learning agent
Q = {} # State-action table
def choose_action(state):
return max(Q.get(state, {}), key=Q.get(state, {}).get, default="random_action")
for episode in range(1000):
state = env.reset()
done = False
while not done:
action = choose_action(state)
next_state, reward, done, _ = env.step(action)
# Update Q-table
Q[state] = Q.get(state, {})
Q[state][action] = Q[state].get(action, 0) + 0.1 * (reward + max(Q.get(next_state, {}).values(), default=0) - Q[state].get(action, 0))
state = next_state
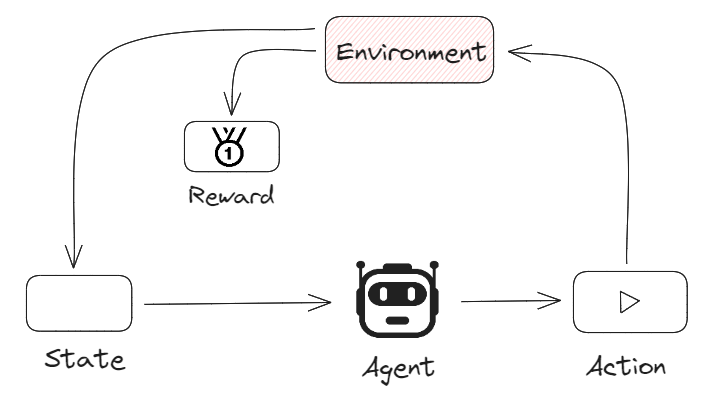
Real-World Applications of Machine Learning
Machine learning’s versatility makes it invaluable across various sectors:
- Healthcare: ML assists with diagnostics, patient risk prediction, and drug discovery.
- Finance: Used for fraud detection, credit scoring, and algorithmic trading.
- Retail: Drives recommendation engines, dynamic pricing, and inventory management.
- Self-driving Cars: ML enables perception, decision-making, and control systems.
For example, spam filters in email platforms leverage classification algorithms to distinguish between spam and genuine emails. Similarly, streaming services use collaborative filtering and deep learning to offer personalized content suggestions.
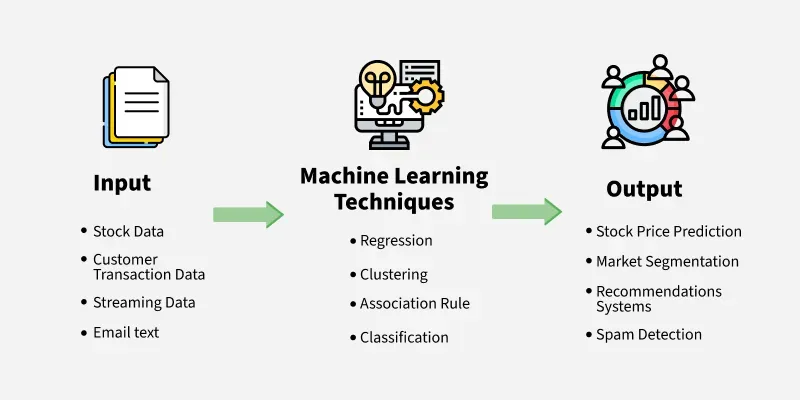
Conclusion
Machine learning stands at the forefront of technological innovation, enabling computers to interpret data, recognize patterns, and make intelligent decisions. Its evolving algorithms and expanding real-world applications promise even greater breakthroughs in the years to come. By understanding the fundamentals and experimenting with practical code, anyone can be part of this exciting journey.
Ready to Start Your ML Journey?
Dive deeper by exploring open datasets, implementing algorithms, and contributing to the dynamic field of machine learning. Your next discovery might power tomorrow’s smart technologies!